I have been toying around with React to refresh my frontend skills (The last time I used full stack seriously was with backbone.js π). While building this app, I wanted to use cookies to store a JWT token from the backend. I did not want the jwt token to be stored in local storage because of the security implications.
Here is my reference app with the problem: The commit with the code that has the problem: https://github.com/minhajuddin/blog-samples/pull/1/commits/adbf276d649af049e56866306983ce653c187c96#diff-f3a80d02e5834a09d590f34b0af1f6c33a4397fd7c4a2eb6847380f228983542R44-R63
Here is the relevant code:
1 | rg.POST("/login", func(c *gin.Context) { |
And, here is the javascript code that makes the request https://github.com/minhajuddin/blog-samples/pull/1/commits/adbf276d649af049e56866306983ce653c187c96#diff-b7726e02edfe1248f6ca02c671344d08f10f81801e755e28c0b8448ce3f50833R27-R34
1 | document.getElementById('login').addEventListener('click', function() { |
And, looking at the network tab in the browser, I see that the cookie is being set, but it is not being sent with the subsequent requests.
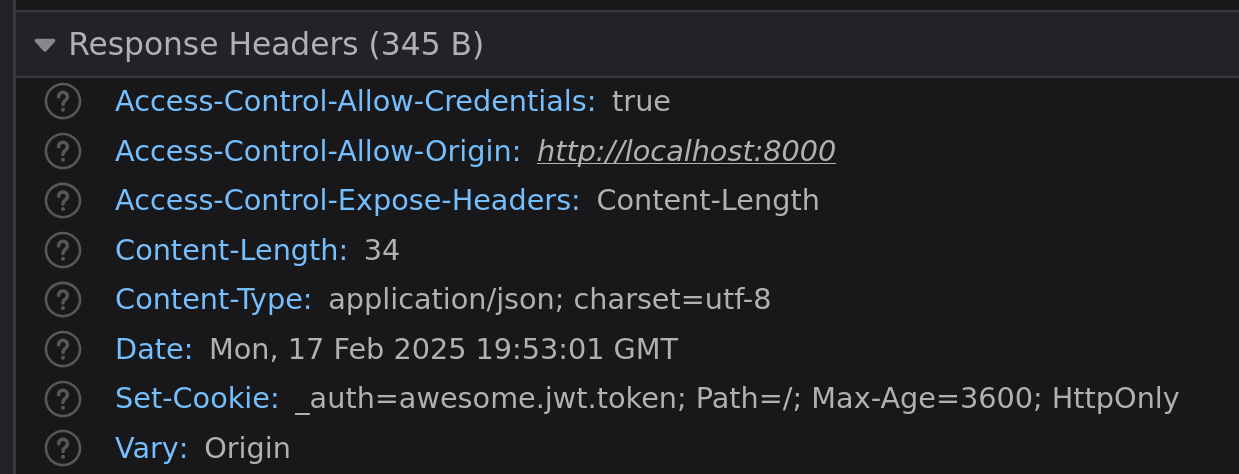
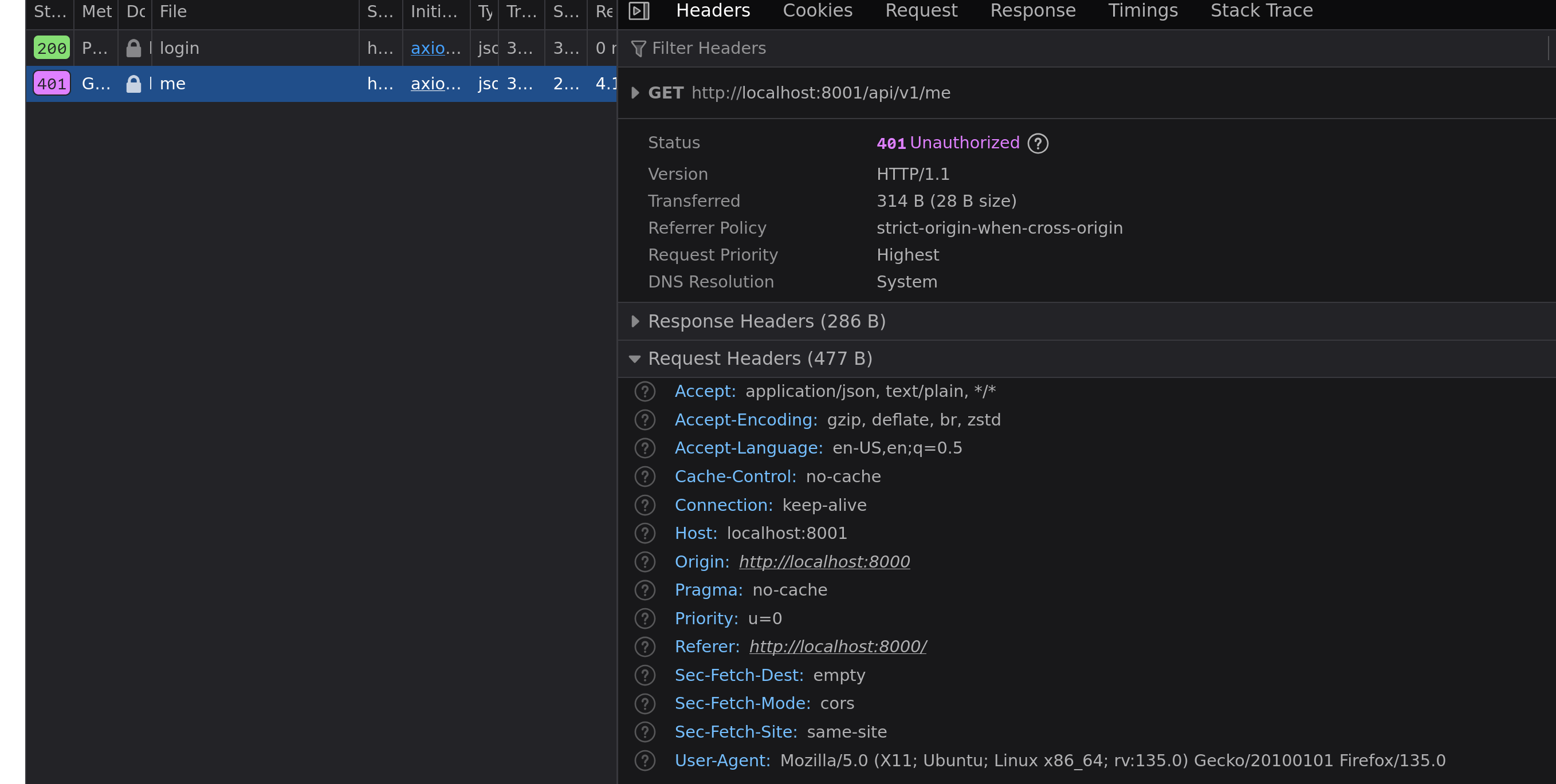
The fix
The problem is that we are not using the withCredentials
option in axios, this is required for cookies to be sent with the request. Letβs fix the code:
1 | document.getElementById('me').addEventListener('click', function() { |
The real fix
This still didnβt fix the problem for me and I was pulling my hair when I finally figured that the withCredentials option needs to be set even for the code that does the authentication. Letβs fix that:
1 | document.getElementById('login').addEventListener('click', function() { |
Now, the cookies are being sent with the request and the backend is able to read the cookie and authenticate the user.
I hope this helps you fix your CORS cookie problems.
Happy hacking! π
P.S: You could also set the withCredentials option globally for axios like so:
1 | axios.defaults.withCredentials = true |